不畏惧失败是创造力的一个基本要素。——<艾尔文·兰德博士>
作者:Ashish Lahoti
译者:cl9000
来源:https://codingnconcepts.com/javascript/console-table-javascript/
在本教程中,我们将学习如何使用 console.table()
高级日志记录方法在控制台中以表格格式显示数据。这对于在 JavaScript
中可视化复杂的数组和对象非常方便。
console.table([])
我们同时使用 console.log()
和 console.table()
记录数字数组,以查看 console.table()
如何很好地打印了一个表来显示数字数组。
1 2 3 4
| var numbers = ["one", "two", "three"];
console.log(numbers); console.table(numbers);
|
Output
1 2 3 4 5 6 7 8
| [ 'one', 'two', 'three' ] ┌─────────┬─────────┐ │ (index) │ Values │ ├─────────┼─────────┤ │ 0 │ 'one' │ │ 1 │ 'two' │ │ 2 │ 'three' │ └─────────┴─────────┘
|
console.table([ [], [], [] ])
与 console.log()
方法相比,使用 console.table()
打印 数组的数组 时,表格格式的可视化更加方便。
1 2 3 4
| var numbers = [["one", "1", "I"], ["two", "2", "II"], ["three", "2", "III"]];
console.log(numbers); console.table(numbers);
|
Output
1 2 3 4 5 6 7 8 9 10
| [ [ 'one', '1', 'I' ], [ 'two', '2', 'II' ], [ 'three', '3', 'III' ] ] ┌─────────┬─────────┬─────┬───────┐ │ (index) │ 0 │ 1 │ 2 │ ├─────────┼─────────┼─────┼───────┤ │ 0 │ 'one' │ '1' │ 'I' │ │ 1 │ 'two' │ '2' │ 'II' │ │ 2 │ 'three' │ '3' │ 'III' │ └─────────┴─────────┴─────┴───────┘
|
console.table(Object)
与数组类似,对象(Objects) 可以使用 console.table()
打印,以表格格式显示。
1 2 3 4 5 6 7 8 9 10
| function Person(firstName, lastName, age) { this.firstName = firstName; this.lastName = lastName; this.age = age; }
var john = new Person("John", "Smith", 41);
console.log(john); console.table(john);
|
Output
1 2 3 4 5 6 7 8
| Person { firstName: 'John', lastName: 'Smith', age: 41 } ┌───────────┬─────────┐ │ (index) │ Values │ ├───────────┼─────────┤ │ firstName │ 'John' │ │ lastName │ 'Smith' │ │ age │ 41 │ └───────────┴─────────┘
|
console.table(Objects[])
与 console.log()
方法相比,使用 console.table()
打印对象数组时,表格格式的可视化更加方便。
1 2 3 4 5 6
| var john = new Person("John", "Smith", 41); var jane = new Person("Jane", "Doe", 38); var emily = new Person("Emily", "Jones", 12);
console.log([john, jane, emily]); console.table([john, jane, emily]);
|
Output
1 2 3 4 5 6 7 8 9 10
| [ Person { firstName: 'John', lastName: 'Smith', age: 41 }, Person { firstName: 'Jane', lastName: 'Doe', age: 38 }, Person { firstName: 'Emily', lastName: 'Jones', age: 12 } ] ┌─────────┬───────────┬──────────┬─────┐ │ (index) │ firstName │ lastName │ age │ ├─────────┼───────────┼──────────┼─────┤ │ 0 │ 'John' │ 'Smith' │ 41 │ │ 1 │ 'Jane' │ 'Doe' │ 38 │ │ 2 │ 'Emily' │ 'Jones' │ 12 │ └─────────┴───────────┴──────────┴─────┘
|
限制列显示
您可以将第二个参数作为要显示的字段数组传递。只有那些列将以表格格式显示。
1
| console.table([john, jane, emily], ["firstName"]);
|
Output
1 2 3 4 5 6 7
| ┌─────────┬───────────┐ │ (index) │ firstName │ ├─────────┼───────────┤ │ 0 │ 'John' │ │ 1 │ 'Jane' │ │ 2 │ 'Emily' │ └─────────┴───────────┘
|
console.table(Object of Objects)
使用 console.table()
很容易 可视化 复杂的嵌套对象
1 2 3 4 5 6 7
| var family = {};
family.mother = new Person("Jane", "Smith", 38); family.father = new Person("John", "Smith", 41); family.daughter = new Person("Emily", "Smith", 12);
console.table(family);
|
Output
1 2 3 4 5 6 7
| ┌──────────┬───────────┬──────────┬─────┐ │ (index) │ firstName │ lastName │ age │ ├──────────┼───────────┼──────────┼─────┤ │ mother │ 'Jane' │ 'Smith' │ 38 │ │ father │ 'John' │ 'Smith' │ 41 │ │ daughter │ 'Emily' │ 'Smith' │ 12 │ └──────────┴───────────┴──────────┴─────┘
|
显示列排序 Sort Column Display
还要注意,当这些表格格式在浏览器控制台中呈现时。您可以单击表列标题来按列排序。
Output
1 2 3 4 5 6 7
| ┌──────────┬─────────────┬──────────┬─────┐ │ (index) │ firstName ▲ │ lastName │ age │ ├──────────┼─────────────┼──────────┼─────┤ │ daughter │ 'Emily' │ 'Smith' │ 12 │ │ mother │ 'Jane' │ 'Smith' │ 38 │ │ father │ 'John' │ 'Smith' │ 41 │ └──────────┴─────────────┴──────────┴─────┘
|
限制列显示 Restrict Column Display
同样,将第二个参数作为要显示的字段数组传递。只有那些列将以表格格式显示。
1
| console.table(family, ["firstName", "age"]);
|
Output
1 2 3 4 5 6 7
| ┌──────────┬───────────┬─────┐ │ (index) │ firstName │ age │ ├──────────┼───────────┼─────┤ │ mother │ 'Jane' │ 38 │ │ father │ 'John' │ 41 │ │ daughter │ 'Emily' │ 12 │ └──────────┴───────────┴─────┘
|
总结
在本教程中,我们了解了 console.table()
如何有助于以表格格式可视化复杂数组和对象,以及如何提供对列进行排序和限制列显示的功能。
所有现代浏览器都支持这种高级日志记录方法,如 chrome、edge、firefox、opera
和safari
。
参考
关注【公众号】,了解更多。
关注【公众号】,了解更多。
关注【公众号】,了解更多。
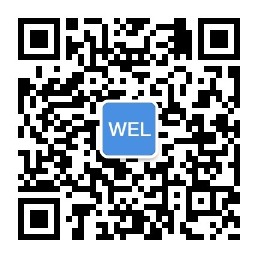
赞赏一下 坚持原创技术分享,您的支持将鼓励我继续创作!