人只有献身于社会,才能找出那短暂而有风险的生命的意义。 ——<阿尔伯特·爱因斯坦>
作者:Ashish Lahoti
译者:cl9000
来源:https://codingnconcepts.com/javascript/how-to-search-array-in-javascript/
在本教程中,我们将学习如何使用 JavaScript
中的不同Array方法在Array中搜索元素。
1. Array.filter()
Array.filter()方法将条件作为函数,并返回满足该条件的元素数组。
例子1
1 2 3 4 5
| const array = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10];
const allEvenNumbers = array.filter(n => n%2==0); const allOddNumbers = array.filter(n => n%2==1); const greaterThanNine = array.filter(n => n>9);
|
例子2
1 2 3 4 5 6 7 8 9 10 11
| const fruits = [ { name: "mango", color: "yellow", calories: 135}, { name: "banana", color: "yellow", calories: 60 }, { name: "apple", color: "red", calories: 65 }, { name: "orange", color: "orange", calories: 50 }, { name: "kiwi", color: "green", calories: 46 }, ];
const yellowFruits = fruits.filter(fruit => fruit.color == "yellow").map(fruit => fruit.name);
const lowCalories = fruits.filter(fruit => fruit.calories <=50).map(fruit => fruit.name);
|
2. Array.find()
Array.find()
方法将条件作为函数,并返回满足该条件的第一个元素。
例子1
1 2 3 4 5
| const array = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10];
const allEvenNumbers = array.find(n => n%2==0); const allOddNumbers = array.find(n => n%2==1); const greaterThanNine = array.find(n => n>9);
|
例子2
1 2 3 4 5 6 7 8 9 10 11 12 13
| const fruits = [ { name: "mango", color: "yellow", calories: 135}, { name: "banana", color: "yellow", calories: 60 }, { name: "apple", color: "red", calories: 65 }, { name: "orange", color: "orange", calories: 50 }, { name: "kiwi", color: "green", calories: 46 }, ];
const yellowFruits = fruits.find(fruit => fruit.color == "yellow"); console.log(yellowFruits);
const lowCalories = fruits.find(fruit => fruit.calories <=50); console.log(lowCalories);
|
3. Array.includes()
Array.includes()方法寻找给定值,并返回true,如果在数组中查找,否则返回false。
例子1
1 2 3 4
| const array = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10];
const includesTen = array.includes(10); const includesTwenty = array.includes(20);
|
例子2
1 2 3 4 5 6 7 8 9 10
| const fruits = [ { name: "mango", color: "yellow", calories: 135}, { name: "banana", color: "yellow", calories: 60 }, { name: "apple", color: "red", calories: 65 }, { name: "orange", color: "orange", calories: 50 }, { name: "kiwi", color: "green", calories: 46 }, ];
const includesMango = fruits.map(fruit => fruit.name).includes("mango"); const includesBlue = fruits.map(fruit => fruit.color).includes("blue");
|
例子3
计算给定字符串中的元音数量:
1 2 3 4
| const vowels = ['a', 'e', 'i', 'o', 'u']; const string = "codingnconcepts"; const countVowels = word.split("").map(char => vowels.includes(char) ? 1 : 0).reduce((a, b) => a + b); console.log(countVowels);
|
4. Array.indexOf()
Array.indexOf()
方法查找给定值,如果在数组中找到,则返回索引,否则返回-1。
例子1
1 2 3 4
| const array = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10];
const indexOfTen = array.indexOf(10); const indexOfTwenty = array.indexOf(20);
|
例子2
1 2 3 4 5 6 7 8 9 10
| const fruits = [ { name: "mango", color: "yellow", calories: 135}, { name: "banana", color: "yellow", calories: 60 }, { name: "apple", color: "red", calories: 65 }, { name: "orange", color: "orange", calories: 50 }, { name: "kiwi", color: "green", calories: 46 }, ];
const indexOfMango = fruits.map(fruit => fruit.name).indexOf("mango"); const indexOfBlue = fruits.map(fruit => fruit.color).indexOf("blue");
|
参考
关注【公众号】,了解更多。
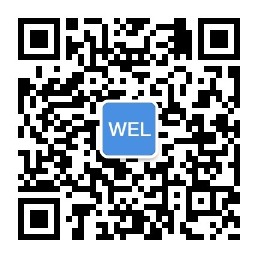
赞赏一下 坚持原创技术分享,您的支持将鼓励我继续创作!